Static Site Generation (SSG) with Next.js
Discover the benefits and features of GMR Transcription services in our detailed review. Learn how to enhance your website with Static Site Generation (SSG) using Next.js for improved performance and scalability.
Static Site Generation (SSG) is a method of building websites where HTML pages are pre-rendered at build time. This approach offers significant benefits, including faster page loads, improved security, and better scalability. In the context of modern web development, Next.js has emerged as a powerful framework that supports SSG, making it easier for developers to create fast and efficient static websites.
Why Choose Next.js for Static Site Generation
Next.js is a popular React framework that simplifies the process of building and deploying static sites. Its key features include:
- Performance: Next.js generates static HTML files for each page, which can be served quickly by a CDN, leading to faster load times.
- SEO: Pre-rendered HTML pages are easily indexed by search engines, enhancing SEO performance.
- Developer Experience: With features like automatic routing, code splitting, and API routes, Next.js offers a streamlined development process.
- Flexibility: Next.js supports both SSG and server-side rendering (SSR), allowing developers to choose the best rendering strategy for their project.
Getting Started with Next.js and SSG
To start using Next.js for static site generation, you need to set up a Next.js project. Here's a step-by-step guide to get you started:
Setting Up the Project
First, create a new Next.js project using the following command:
npx create-next-app my-static-site
Navigate to the project directory:
cd my-static-site
Creating Static Pages
In Next.js, pages are created by adding files to the pages
directory. To generate a static page, simply create a new file in this directory. For example, to create an About
page, add an about.js
file in the pages
directory:
// pages/about.js export default function About() { return ( <div> <h1>About Us</h1> <p>Welcome to our static site powered by Next.js!</p> </div> ); }
Next.js will automatically generate a static HTML page for this route during the build process.
Fetching Data for Static Pages
Next.js allows you to fetch data at build time using the getStaticProps
function. This is particularly useful for creating pages with dynamic content. Here's an example of how to use getStaticProps
:
// pages/blog.js import fs from 'fs'; import path from 'path'; export async function getStaticProps() { const filePath = path.join(process.cwd(), 'data', 'posts.json'); const jsonData = fs.readFileSync(filePath); const posts = JSON.parse(jsonData); return { props: { posts, }, }; } export default function Blog({ posts }) { return ( <div> <h1>Blog</h1> <ul> {posts.map((post) => ( <li key={post.id}>{post.title}</li> ))} </ul> </div> ); }
In this example, data is fetched from a local JSON file at build time and passed as props to the Blog
component.
Generating Static Paths
For dynamic routes, Next.js provides the getStaticPaths
function. This function is used to specify which paths should be pre-rendered at build time. Here's an example:
In this example, getStaticPaths
generates the paths for each post, and getStaticProps
fetches the data for each individual post based on the path.
Optimizing Performance
To further optimize the performance of your static site, consider the following tips:
- Use a CDN: Serve your static files from a Content Delivery Network (CDN) to reduce latency and improve load times.
- Optimize Images: Use the Next.js
Image
component to optimize and lazy-load images. - Code Splitting: Next.js automatically splits your code into smaller bundles, ensuring that users only download the necessary code for each page.
- Prefetch Links: Use the
prefetch
attribute on the Next.jsLink
component to prefetch linked pages in the background, making navigation faster.
Deploying Your Static Site
Once your site is ready, you can deploy it to various platforms. Some popular options include:
- Vercel: The creators of Next.js, Vercel offers seamless integration with Next.js, making deployment straightforward.
- Netlify: Another popular platform for deploying static sites, with excellent support for Next.js.
- GitHub Pages: Host your static site directly from a GitHub repository.
To deploy to Vercel, you can use the following command:
npx vercel
Follow the prompts to deploy your site. Vercel will handle the rest, including setting up a custom domain if desired.
Static Site Generation with Next.js offers a powerful and efficient way to build modern websites. By pre-rendering pages at build time, you can achieve fast load times, enhanced SEO, and a better overall user experience. With its robust feature set and excellent developer experience, Next.js is an excellent choice for static site generation. Whether you're building a simple blog or a complex web application, Next.js provides the tools and flexibility needed to create high-performance static sites.
What's Your Reaction?
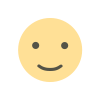




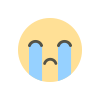
